Mint and Transfer ERC721/NFTS gaslessly with Biconomy SDK
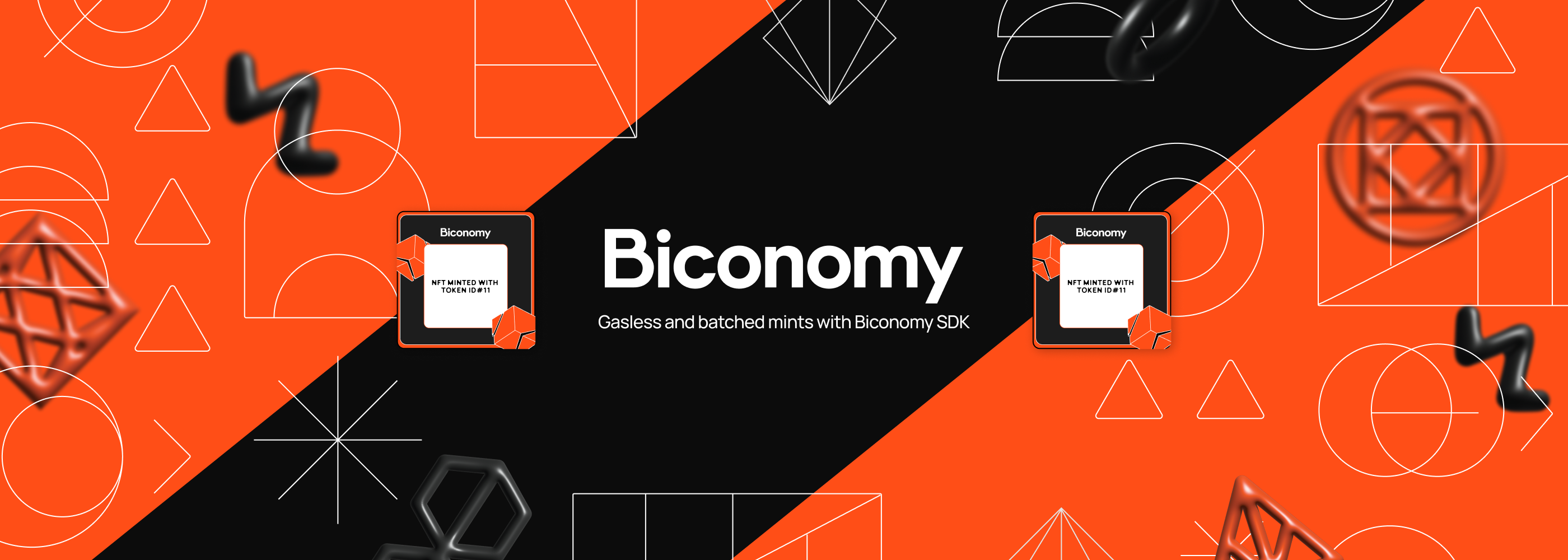
💡 In this tutorial, we will interact with ERC721 Contract deployed on polygon Mumbai. We will mint multiple NFTs in our smart account address batched together and sent via paymaster. Once minted, we will send a transaction of transferring this from our address to another user's address. We shall be using Hardhat,React JS and Vite in this project.
1.Creating and configuring a Hardhat project
2. Create a smart contract of ERC721 and add SVG code
3. Deploying and verifying your contract to testnet
4. Setting up biconomy dashboard to setup gasless transactions
5. Configuring frontend for the dApp using Vita and React
6. Allow social logins for this dApp
7. Setting backend logic for minting and transferring NFTs by Token ID
Creating and configuring a Hardhat project
- Create a new folder with the desired name of your project dApp in VS Code .
- Once created, create a sub folder in root directory of your project named Contract. Once created, navigate to this directory in your terminal and then do cd contract.
- Then, start installing hardhat for your project in that folder. Follow the following commands : npm install --save-dev hardhat
- In the same directory where you installed Hardhat run: npx hardhat
- You will be given three options to create your project, elect Create a TypeScript project with your keyboard and hit enter.
- This will create a folder within contract folder and have hardhat.config.ts as well.
- Now, we would install @nomicfoundation/hardhat-toolbox, which has everything you need for developing smart contracts.
- npm install --save-dev @nomicfoundation/hardhat-toolbox
/
If you select Create a Typescript project, a simple project creation wizard will ask you some questions like if you want to have .gitignore etc. After that, the wizard will create some directories and files and install the necessary dependencies.
The initialized project has the following structure:
- contracts/
- scripts/
- test/
- hardhat.config.ts
These are the default paths for a Hardhat project
contracts/ is where the source files for your contracts should be.
test/ is where your tests should go.
scripts/ is where simple automation scripts go.
Creating Smart Contract for ERC721 Token
Once you have got the hardhat installed, then we will have add our ERC721 Contract . For this, as per the structure, go to cd contract and then go to cd contracts
- Name your contract with .sol extension just like I have done here.
The structure of the file is going to look like such -
.png)
License
At the start of your smart contract, you can declare a license. In this case, we will leave this as UNLICENSED as we are not too concerned about what this code is used for.
Mention Compiler Version
Make sure the solidity version declared in the contract matches your compiler version
Let's work on our contract :
1. We would need to import these contracts in our main contract.
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721Enumerable.sol";
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
import "@openzeppelin/contracts/utils/Base64.sol";
import "@openzeppelin/contracts/utils/Strings.sol";
- ERC721.sol: This contract implements the ERC721 standard, which defines a non-fungible token (NFT) interface. NFTs are unique tokens that represent ownership or proof of authenticity of a specific asset, such as digital artwork or collectibles.
- ERC721Enumerable.sol: This contract extends the ERC721 standard and adds functionality to enumerate and iterate over a collection of NFTs. It allows for querying the total supply, getting the NFT at a specific index, and finding the index of a given NFT token ID.
- ERC721URIStorage.sol: This contract extends the ERC721 standard and provides functionality to store and retrieve the metadata associated with each NFT token. The metadata typically includes information such as the name, description, and image URL of the NFT.
- Ownable.sol: This contract provides a basic access control mechanism, allowing the contract owner to restrict certain functions to be executed only by the owner. It adds a modifier that can be applied to functions, ensuring that only the owner can call those functions.
- Counters.sol: This contract provides a utility for managing and tracking numerical counters. It includes functions to increment and decrement counter values and retrieve the current count.
- Base64.sol: This contract provides functions to encode and decode data using the Base64 encoding scheme. Base64 encoding is commonly used to convert binary data into a textual format for transmission or storage.
- Strings.sol: This contract provides utility functions for manipulating strings, such as concatenation, length calculation, and converting integers to strings.
2. Writing NFT contract:
Here is an explanation to what is happening in the above solidity code.
- Counters: Counters.Counter is a struct defined in the Counters.sol library. It is used to keep track of the current token ID. _tokenIdCounter is an instance of this struct and is declared as private.
- Constructor: The constructor function is called when the contract is deployed. It initializes the contract and sets the name and symbol of the NFT contract using the ERC721 constructor.
- generateSVG: This function takes a tokenId as input and returns an SVG image as a string. It concatenates multiple SVG elements using string interpolation and encodes the resulting SVG as a Base64 string.
Now let's work on next set of our solidity code:
- getNFTID: This function takes a tokenId as input and converts it to a string using the toString() function from the Strings library. It returns the resulting string.
- getTokenURI: This function takes a tokenId as input and returns the token's metadata URI as a string. It generates the JSON metadata object using the generateSVG function and concatenates it with other metadata information, such as the name and description. The resulting JSON metadata object is encoded as a Base64 string.
Now, we will add our mint , transferNFT and getOwnedNFT function :
- mint: This function is used to mint a new NFT. It increments the _tokenIdCounter and retrieves the current tokenId. Then, it calls _safeMint (inherited from ERC721) to create a new NFT with the given tokenId. Finally, it sets the token's URI using _setTokenURI, passing the tokenId and the result of getTokenURI.
- transferNFT: This function is used to transfer ownership of an NFT to a specified recipient. It checks various conditions, such as the existence of the token, the caller's ownership or approval, and the validity of the recipient's address. If all conditions are met, it calls safeTransferFrom (inherited from ERC721) to perform the transfer.
- getOwnedNFTs: This function returns an array of token IDs representing the NFTs owned by the caller. It retrieves the balance of the caller's NFTs using balanceOf, creates an array with the appropriate length, and populates it with the token IDs using tokenOfOwnerByIndex.
Now, our last step would be adding overrides functions:
Overrides and Interactions with Inherited Contracts: The contract includes several functions that override or interact with functions inherited from the ERC721, ERC721Enumerable, and ERC721URIStorage contracts. These functions include _beforeTokenTransfer, _burn, tokenURI, and supportsInterface. These overrides ensure the correct behavior and compatibility with the underlying contracts.
The override keyword is used in Solidity to explicitly indicate that a function is intended to override a function from a base contract. Let's go through each of these functions:
- _beforeTokenTransfer(address from, address to, uint256 tokenId, uint256 batchSize) internal override(ERC721, ERC721Enumerable): This function overrides the _beforeTokenTransfer function defined in both the ERC721 and ERC721Enumerable contracts. It is called internally before a token transfer occurs. By overriding this function, you can add custom logic that should be executed before a token is transferred.
- _burn(uint256 tokenId) internal override(ERC721, ERC721URIStorage): This function overrides the _burn function defined in both the ERC721 and ERC721URIStorage contracts. It is responsible for burning (deleting) a token with the given tokenId. By overriding this function, you can add additional actions or restrictions before the token is burned.
- tokenURI(uint256 tokenId) public view override(ERC721, ERC721URIStorage) returns (string memory): This function overrides the tokenURI function defined in both the ERC721 and ERC721URIStorage contracts. It retrieves the metadata URI associated with a given token tokenId. By overriding this function, you can customize how the metadata URI is generated or retrieved.
Here is the entire contract code :
Deploying and verifying your contract to testnet
1. Creating .env file inside the contract folder :
In order to deploy and verify our above smart contract, we would need to create .env file in the below format.
In PRIVATE_KEY enter the private key of your Metamask wallet address on Polygon Mumbai Network that would cover the initial cost of deployment. Make sure to have some funds in matic in the same wallet. You can use faucets available here - https://faucet.polygon.technology
POLYGONSCAN_API_KEY enter the API Key that you can get from https://polygonscan.com/myapikey You can go to your Account Dashboard, click on the navigation tab labelled API-KEYs.From there, you may click on Add to create a new key and give a name to your project. Each PolygonScan account is limited to creating 3 keys at any one time.
Let's move on to next steps.
2. Deploy.ts for NFT Contract
First, we would be deploying our smart contract on polygon Mumbai network and verify on the testnet. For this, we would need to look at our deploy.ts first which is in the sub folder of scripts.
- The main function is defined as an asynchronous function, which means it can use the await keyword to wait for promises to resolve. This function will be the entry point for the script.
- Inside the main function, it calls ethers.getContractFactory("NFTContract") to retrieve the contract factory for a smart contract named "NFTContract". This assumes that you have a contract named "NFTContract" defined in your project.
- The script then deploys the contract by calling nft.deploy(). This deploys an instance of the contract to the Ethereum network.
- After deploying the contract, it waits for the deployment to complete by calling await nft.deployed().
- Finally, it logs a message to the console, indicating that the NFT contract has been deployed and displays the contract's address. The message also mentions the usage of the Biconomy SDK for the demo, indicating that the Biconomy SDK is being used with this contract.
- The main function is invoked by calling main().catch((error) => { ... }). This ensures that any errors that occur during the execution of the main function are caught and logged to the console. The process.exitCode = 1 line sets the exit code of the script to 1 in case of an error.
Execute this command in your terminal to deploy the contract :
You should get something like this with a contract address deployed on testnet.
This is what I get in terminal when I run the above command :

3. Verifying the smart contract post deployment :
Next step would be verifying the contract address, use the following command -
This will verify your contract on Polygonscan and give you a verified address and a link in terminal which you can check on polygon scan. It would look something like this :
My contract address has already been verified !
.png)
Now, we can add this verified contract address on Biconomy dashboard. Let's see how.
Setting up Biconomy Dashboard for Gasless Transactions
We will use the verified address in the dashboard for whitelising the address which will allow us to set up a gas tank and do gasless transactions.
- Visit Biconomy's new dashboard
- You will get three sign up options, you can use magic link or github to login.
- Once logged in, you will be able to see this -
.png)
- Now we will go on ADD NEW PAYMASTER to setup for the contract.
- You will see a popup to add the NAME and CHAIN . Add the name of paymaster and the chain on which you have deployed the contract.
.png)
- The next step is going to be adding or whitelisting our smart contracts. All you need to do is go to “Policies” and click on “Add you first smart contract”. After this the ABI will be fetched automatically. You can choose the WRITE METHODS as well and it will show under active contract.
.png)
- After you have succesfully setup your paymaster details, you will see API KEY on dashboard which will be need to add in our .env file for frontend later.
- Now, click on gas tank. You can recharge the gas tank from your EOA/Metamask wallet. Make sure to connect it to dashboard, enter the amount you would like to refill with and then click on depost. Finally, you will get a popup to add the matic funds in gas tank.
Voila ! You have activated the gas tank that will be sponsoring the gas fees for your users.
You can check out this documentation for more information around Biconomy Dashboard - https://docs-gasless.biconomy.io/guides/biconomy-dashboard
Configuring Frontend for your dApp using Vite
For this project we are using Vita + React. For this, we will go to root repository, and in the terminal, enter the following commands to install site for setting up frontend-
With NPM
With Yarn:
You can check out this documentation as well - https://vitejs.dev/guide/#scaffolding-your-first-vite-project
Install the following dependencies:
We will use these tools to build out our front end. In addition, lets also install the following:
Once we have installed all the required dependencies, let's configure our vite.config.ts file.
Now, let's create another .env file where we would be adding the Biconomy dApp API key from dashboard, and the verified contract address as well.
Allowing Social Logins for the dApp using Biconomy SDK
Now, that we are done with configuration of frontend, let's move on to some actual use case of having social logins via web3auth and on top of that having smart contract wallet implementation.
For this, I am creating a separate sub folder called src inside the root folder of dapp. This folder will contain tsx and css files.
Here is how the structure looks like for me :
.png)
- Main.tsx :
This code sets up a basic React application with a logo image, headings, an 'App' component, and a footer. It likely represents a web page or a part of a web application that demonstrates the usage of the Biconomy SDK for batched transactions.
Allowing Social Logins for the dApp using Biconomy SDK
To add social logins, let's go to App.tsx file in the src folder.
We would import the following :
We are using the css files from app.css and then from web3auth package. You can make changes on frontend according to your requirements.
Here is the breakdown for the imports we are using :
useState, useEffect, useRef: React hooks for managing component state and lifecycle.
SocialLogin from @biconomy/web3-auth: A class from Biconomy SDK that allows you to leverage Web3Auth for social logins.
ChainId from @biconomy/core-types: An enumeration of supported blockchain networks.
ethers: A library for interacting with Ethereum.
SmartAccount from @biconomy/smart-account: A class from Biconomy SDK that will allow us to create smart accounts and interact with our contract with them.
Moving on, lets define our state variables:
1. const [smartAccount, setSmartAccount] = useState<SmartAccount | null>(null)
- This line declares a state variable called smartAccount using the useState hook. It initializes the state with a value of null and provides a setter function setSmartAccount to update the state value. The type annotation <SmartAccount | null> indicates that the state variable can hold values of type SmartAccount or null.
2. const [interval, enableInterval] = useState(false)
- This line declares another state variable called interval and its setter function enableInterval. It initializes the state with a value of false. This state variable is likely used to control the enabling or disabling of an interval mechanism.
3. const sdkRef = useRef<SocialLogin | null>(null)
- This line declares a ref called sdkRef using the useRef hook. The ref is initialized with a value of null, and its type annotation <SocialLogin | null> suggests that it can reference an instance of SocialLogin or null.
4. const [loading, setLoading] = useState<boolean>(false)
- This line declares a state variable called loading and its setter function setLoading. It initializes the state with a value of false. This state variable is likely used to indicate the loading state of a component or operation.
5. const [provider, setProvider] = useState<any>(null)
- This line declares a state variable called provider and its setter function setProvider. It initializes the state with a value of null. The type annotation any indicates that the state variable can hold values of any type.
6. const [acct, setAcct] = useState<any>(null)
- This line declares a state variable called acct and its setter function setAcct. It initializes the state with a value of null. Similar to the previous line, the type annotation any suggests that this state variable can hold values of any type.
Here we have some state that will be used to track our smart account that will be generated with the sdk, an interval that will help us with checking for login status, a loading state, provider state to track our web3 provider and a reference to our Social login sdk.
Next let's add a useEffect hook:
This use effect will be triggered after we open our login component, which we'll create a function for shortly. Once a user opens the component it will check if a provider is available and run the functions for setting up the smart account.
Now our login function:
The login function is an asynchronous function that handles the login flow for the application. Here's a step-by-step explanation:
- SDK Initialization: The function first checks if the sdkRef object (which is a reference to the Biconomy SDK instance) is null. If it is, it means that the SDK is not yet initialized. In this case, it creates a new instance of SocialLogin (a Biconomy SDK component), whitelists a local URL ( [http://localhost:5173/](<http://localhost:5173/>) ), and initializes the SDK with the Polygon Mumbai testnet configuration and the whitelisted URL. After initialization, it assigns the SDK instance to sdkRef.current.
- Provider Check: After ensuring the SDK is initialized, the function checks if the provider of the sdkRef object is set. If it is not, it means the user is not yet logged in. It then shows the wallet interface for the user to login using sdkRef.current.showWallet(), and enables the interval by calling enableInterval(true). This interval (setup in a useEffect hook elsewhere in the code) periodically checks if the provider is available and sets up the smart account once it is.
- Smart Account Setup: If the provider of sdkRef is already set, it means the user is logged in. In this case, it directly sets up the smart account by calling setupSmartAccount().
In summary, the login function handles the SDK initialization and login flow. It initializes the SDK if it's not already initialized, shows the wallet interface for the user to login if they're not logged in, and sets up the smart account if the user is logged in.
Note - It is important to make sure that you update the whitelist URL with your production url when you are ready to go live!
Now, let's setup our smart account using the SDK :
The setupSmartAccount function is an asynchronous function used to initialize a smart account with Biconomy and connect it with the Web3 provider. Here's a step-by-step explanation of what it does:
- Check Provider Availability: The function first checks if the provider of the sdkRef object is available. If not, it immediately returns and the rest of the function is not executed. The sdkRef object refers to the Biconomy SDK instance that was stored using the useRef React Hook.
- Hide Wallet: If the provider is available, it hides the wallet interface using sdkRef.current.hideWallet().
- Set Loading Status: It then sets the loading state to true by calling setLoading(true). This could be used in the UI to show a loading spinner or other loading indicators.
- Create Web3 Provider: It creates a new Web3 provider using the ethers library and the provider from sdkRef. This provider is then saved in the state by calling setProvider(web3Provider).
- Create and Initialize Smart Account: It then creates a new SmartAccount object using the Web3 provider and a configuration object. This configuration object sets the active network to Polygon Mumbai, the supported networks, and the network configuration, including the chain ID and the Biconomy API key. After creating the smart account, it initializes it by calling smartAccount.init(). This is an asynchronous operation, hence the await keyword.
- Save Smart Account and Update Loading Status: After the smart account is initialized, it is saved in the state by calling setSmartAccount(smartAccount). The loading status is then set to false by calling setLoading(false).
- Error Handling: If any error occurs during the creation or initialization of the smart account, it is caught in the catch block and logged to the console.
So, in summary, the setupSmartAccount function checks the availability of the Biconomy provider, hides the wallet interface, sets up a Web3 provider, creates and initializes a smart account, and then saves this account and the Web3 provider in the state. If any error occurs during this process, it is logged to the console.
Finally our last function will be a logout function:
The logout function is an asynchronous function that handles the logout flow for the application. Here's a breakdown of its functionality:
- Check SDK Initialization: The function first checks if the sdkRef object (which is a reference to the Biconomy SDK instance) is null. If it is, it means that the SDK is not yet initialized. In this case, it logs an error message and returns immediately without executing the rest of the function.
- Logout and Hide Wallet: If the SDK is initialized, it logs the user out by calling sdkRef.current.logout(). This is an asynchronous operation, hence the await keyword. It then hides the wallet interface by calling sdkRef.current.hideWallet().
- Clear Smart Account and Interval: After logging the user out and hiding the wallet, it clears the smart account by calling setSmartAccount(null), and disables the interval by calling enableInterval(false).
In summary, the logout function checks if the SDK is initialized, logs the user out and hides the wallet if it is, and then clears the smart account and disables the interval. If the SDK is not initialized, it logs an error message and does not execute the rest of the function.
Finally, you can add this component in your App.tsx to show on load on frontend.
The entire code would look something like this :
To give you an overview, this is how your localhost is going to look like -
.png)
This is how the login popup would look like when you click on “Login to get your scw address”
.png)
Now, let's move on to setting up our minter.tsx and enabling gasless transactions.
Setting backend logic for minting and transferring NFTs by Token ID
Working on vite-env.d.ts configuration :
One important configuration to understand in vite-env.d.ts that we are using TypeScript interfaces for the ImportMetaEnv and ImportMeta objects, which are used to provide type information for the environment variables available in a Vite application.
- ImportMetaEnv is an interface that represents the shape of the environment variables object (import.meta.env). In this example, it includes a single property VITE_CUSTOM_ENV_VARIABLE of type string. You can add more properties to represent additional environment variables used in your application.
- ImportMeta is an interface that represents the shape of the import.meta object. It includes a single property env of type ImportMetaEnv, which provides access to the environment variables defined in the ImportMetaEnv interface.
These interfaces are used to provide type information for the environment variables and import.meta object in a Vite application. By using these interfaces, TypeScript can provide autocompletion, type checking, and type inference for environment variables accessed through import.meta.env in your code.
Now, let move to our src file, we have a sub-folder called component, where we will add our minter.tsx file.
This is where the actual magic begins !
We will divide the transactions in two parts :
- Minting ERC721 tokens to smart contract wallet address - this function will be known as mintNftAsBalance Function
- Minting and Transferring NFTs from the owned NFTs by TokenID to a different address- this function will be known as mintandTransferNFT Function
In both approach, we will check our NFTs shown on OpenSea post minting.
To begin with :
As we would be calling import abi from "../utils/abi.json", we would need to create a separate folder called utils, under which we add abi.json.
Import the following :
Load the interface props:
Now build the components:
Calling the Verified contract address of NFT Contract :
This useEffect hook is called when the component mounts (initial render) due to the empty dependency array []. It means that it will only run once, similar to the behavior of componentDidMount in class components.
>>First useEffect :
Inside the useEffect hook, two functions are called: getNFTCount() and getNFTIndexes(). These functions are likely defined elsewhere in the component or imported from other modules. The purpose of these functions is not apparent from the given code snippet, but they are likely responsible for fetching data related to NFT counts and indexes.
>>Second useEffect :
Inside the useEffect hook, the setNftsAsBalanceCount function is called with the nftCount value as an argument. This suggests that setNftsAsBalanceCount is a setter function for a state variable called nftsAsBalanceCount. It updates the nftsAsBalanceCount state variable with the current value of nftCount whenever nftCount changes.
The code snippet defines an asynchronous function getNFTCount() that performs the following actions:
- Creates a new instance of the ethers.Contract class using the provided nftAddress, abi, and provider variables. The nftAddress likely represents the address of an NFT contract on the Ethereum network, abi represents the contract's ABI (Application Binary Interface), and provider is an instance of the ethers.js provider.
- Sets the NFT contract instance by calling setNFTContract(contract). The setNFTContract function is likely a setter function for a state variable that holds the NFT contract instance.
- Calls the balanceOf() function on the NFT contract, passing smartAccount.address as an argument. This function likely retrieves the balance (number of NFTs) associated with the smartAccount address.
- Sets the NFT count by calling setNFTCount(count.toString()). The setNFTCount function is likely a setter function for a state variable that holds the count of NFTs. The count is first converted to a string before being set.
Overall, the getNFTCount() function retrieves the NFT count associated with a specific address (smartAccount.address) by interacting with an NFT contract deployed at nftAddress. It sets the NFT contract instance and the NFT count using respective setter functions, which are likely used to update the component's state and trigger re-renders.
The code snippet defines an asynchronous function getNFTIndexes() that performs the following actions:
- Creates a new instance of the ethers.Contract class using the provided nftAddress, abi, and provider variables. This is similar to what was done in the getNFTCount() function.
- Sets the NFT contract instance by calling setNFTContract(contract). This is likely a setter function for a state variable that holds the NFT contract instance.
- Calls the balanceOf() function on the NFT contract, passing smartAccount.address as an argument. This retrieves the balance (number of NFTs) associated with the smartAccount address and stores it in the countdata variable.
- Initializes an empty array nftIndexes to store the token indexes associated with the smartAccount address.
- Starts a loop using a for statement, iterating from 0 to countdata (exclusive).
- Inside the loop, it calls the tokenOfOwnerByIndex() function on the NFT contract, passing smartAccount.address and i as arguments. This retrieves the token index (unique identifier) for the NFT owned by the smartAccount at the current index i.
- Converts the index to a string and pushes it to the nftIndexes array using nftIndexes.push(index.toString()).
- After the loop completes, it sets the NFT indexes by calling setNFTIndexes(nftIndexes). This is likely a setter function for a state variable that holds the array of NFT indexes.
Overall, the getNFTIndexes() function retrieves the indexes of NFTs owned by a specific address (smartAccount.address) by interacting with an NFT contract deployed at nftAddress. It sets the NFT contract instance, iterates over the NFTs, retrieves their indexes, and stores them in the nftIndexes array. Finally, it sets the NFT indexes using a setter function, which is likely used to update the component's state and trigger re-renders.
1. Minting ERC721 tokens to smart contract wallet address :
- Creates a new instance of the ethers.Contract class using the provided nftAddress, abi, and provider variables. This is similar to what was done in the previous functions.
- Sets the NFT contract instance by calling setNFTContract(contract). This is likely a setter function for a state variable that holds the NFT contract instance.
- Calls the populateTransaction.mint() function on the NFT contract twice to generate two separate minting transactions. The mint() function is likely responsible for creating a new NFT.
- Creates tx1 and tx2 objects that represent Ethereum transactions to mint the NFTs. Each transaction specifies the destination address (nftAddress) and the transaction data obtained from the corresponding minting transaction (mintTx1.data and mintTx2.data).
- Uses the smartAccount to send a batch of transactions to mint the NFTs. It calls the sendTransactionBatch() function on the smartAccount object and passes an arrayof transactions ([tx1, tx2]) as the transactions parameter.
- Waits for the response of the minting transaction by calling await mintResponse.wait(). This ensures that the transaction is mined and included in a block.
- Prints the transaction hash of the minting transaction by logging console.log('Tx Hash', txReciept.transactionHash).
- Logs the mintResponse object to the console, which likely contains information about the minting transaction and its status.
- Calls the getNFTCount() function, which is likely responsible for updating the NFT count by fetching the latest count after the minting operation.
- If any errors occur during the minting process, they are caught and logged to the console using console.log(error).
Overall, the mintNftAsBalance() function mints two NFTs by interacting with an NFT contract deployed at nftAddress. It generates the minting transactions, sends them as a batch using the smartAccount object, waits for the transaction confirmation, and updates the NFT count by calling getNFTCount().
2. Minting and Transferring NFTs from the owned NFTs by TokenID to a different address
The code snippet provided includes a function named mintAndTransferNft. Here's an explanation of what each function call inside this function is doing:
- ethers.Contract: This is a constructor function from the ethers.js library. It creates a new instance of a smart contract by providing the contract address (nftAddress), the contract ABI (abi), and a provider object (provider).
- setNFTContract: This is a function that sets the NFTContract state variable. It is likely a state setter function provided by a React hook, updating the value of NFTContract with the newly created contract instance.
- document.getElementById("nft-select"): This retrieves an HTML element with the id "nft-select" from the DOM. It is likely an HTML select element used to select an NFT.
- parseInt(nftSelect.value, 10): This converts the value of the selected option in the HTML select element to an integer. It is likely used to retrieve the selected NFT ID.
- console.log('Balance:', nftsAsBalanceCount): This logs the value of nftsAsBalanceCount to the console, displaying the NFT balance.
- console.log('Selected NFT ID:', selectedNftId): This logs the value of selectedNftId to the console, displaying the selected NFT ID.
- contract.populateTransaction.mint(): This is a function call that generates a transaction object for minting a new NFT. It uses the mint() function of the contract object.
- contract.populateTransaction["safeTransferFrom(address,address,uint256)"]: This is a dynamic function call that generates a transaction object for transferring an NFT to a recipient address. It uses the safeTransferFrom function of the contract object and provides the sender address (smartAccount.address), recipient address (recipientAddress), and selected NFT ID (selectedNftId) as arguments.
- smartAccount.sendTransactionBatch: This is a function call that sends a batch of transactions using the sendTransactionBatch method of the smartAccount object. It takes an array of transaction objects (tx1 and tx2) as an argument.
- transferResponse.wait(): This waits for the completion of the transaction batch sent in the previous step. It returns a promise that resolves when the transaction is confirmed.
- console.log({ transferResponse }): This logs the transferResponse object to the console. It likely contains information about the transaction batch's response, such as transaction hashes or status.
These functions collectively perform the minting and transfer of an NFT, utilizing a smart contract, selected NFT ID, and recipient address.
Let's add a popup message for our successful transaction :
Moving on, lets add another set of function to update the balance and the drop down menu of the NFT IDs that is available for transfer :
To breakdown why and what is happening here -
- const count = await contract.balanceOf(smartAccount.address);: This line retrieves the balance of NFTs owned by the smartAccount.address address. It calls the balanceOf function of the contract object, passing the account address as an argument. The returned count is stored in the count variable.
- setNFTCount(count.toString());: This line converts the count to a string and sets it as the new value for the NFTCount state variable. It is likely a state setter function provided by a React hook, updating the NFT count in the component's state.
- const balance = parseInt(count.toString());: This line converts the count to an integer using parseInt and stores it in the balance variable.
- const updatedNFTIndexes = [];: This line initializes an empty array updatedNFTIndexes that will store the updated token ID list.
- if (selectedNftId <= balance) { ... }: This condition checks if the selectedNftId is less than or equal to the balance. If true, it means the selected NFT ID is within the range of the owned NFTs.
- Inside the if block, there is a for loop that iterates over the NFTs owned by the smartAccount.address. It starts from i = 0 and continues until i is less than balance.
- Within the for loop, const index = await contract.tokenOfOwnerByIndex(smartAccount.address, i); retrieves the token ID at the given i index using the tokenOfOwnerByIndex function of the contract object. The token ID is stored in the index variable.
- updatedNFTIndexes.push(index.toString()); converts the index to a string and adds it to the updatedNFTIndexes array.
- After the loop ends, setNFTIndexes(updatedNFTIndexes); sets the updatedNFTIndexes array as the new value for the NFTIndexes state variable. It is likely another state setter function provided by a React hook, updating the token ID list in the component's state.
- The code is wrapped in a try-catch block to catch any errors that occur during the process. If an error occurs, it will be logged to the console using console.log(error).
Note :
In this flow is that the reason why we are minting from the smart account address and adding it as a balance is because we need to hold the tokens in our smart account address that we are trying to send or transfer, otherwise we may fail the transaction and get Call gas estimation error . This means that, until and unless you don't have funds/tokens, you can't send an operation from the smart account for same.
- Checking the minted and transfer NFTs on OpenSea testnet :
- nftURL is a string that represents the URL of the OpenSea marketplace for the NFT associated with the smartAccount.address. It uses template literals to interpolate the smartAccount.address into the URL. This URL is likely used to display the NFT on OpenSea for the account associated with smartAccount.address.
- transferURL is a string that represents the URL of the OpenSea marketplace for the recipient address (recipientAddress). Similar to nftURL, it uses template literals to interpolate the recipientAddress into the URL. This URL is likely used to display the recipient's OpenSea profile or collection on OpenSea.
The URLs are constructed using the base URL for the OpenSea testnet marketplace (https://testnets.opensea.io/) followed by the corresponding Ethereum addresses (smartAccount.address and recipientAddress) to navigate to the respective pages on OpenSea.
- Calling the rest of the code in Minter.tsx :
We are having two Container Box elements which are conditionally rendered based on the value of nftsAsBalanceCount :
- The nftIndexes array is sorted in ascending order using the sort() method. The sorting is based on comparing the elements an and b using the arrow function (a, b) => a - b. This will result in sortedIndexes containing the sorted array of NFT indexes.
- In container-box-1, the content is conditionally rendered based on the condition nftsAsBalanceCount >= 2. Here's how it works:
- If nftsAsBalanceCount is greater than or equal to 2, the condition is true. In this case, the first block is rendered.
- The paragraph <p> element displays the message "You have {nftCount} NFTs in your balance," where {nftCount} is the value of the nftCount variable.
- There are two buttons rendered: "Mint NFTs as balance" and "View minted on SCW address on OpenSea." The onClick event handlers are assigned to the corresponding functions mintNftAsBalance and () => window.open(nftURL, '_blank').
- If nftsAsBalanceCount is less than 2, the condition is false. In this case, the second block is rendered.
- The paragraph <p> element displays the message "Please Mint more NFTs to add as balance for transfer."
- There is a button rendered with the label "Mint NFTs as balance," and the onClick event handler is assigned to the mintNftAsBalance function.
In container-box-2, the content is conditionally rendered based on the condition nftsAsBalanceCount >= 2. Here's how it works:
- If nftsAsBalanceCount is greater than or equal to 2, the condition is true. In this case, the entire block within parentheses is rendered.
Inside the div with the className="container-box-2", there are two sections:
- The first section includes an input field labeled "Recipient Address." It is an <input> element that binds its value to the recipientAddress state variable and updates it using the onChange event handler.
- The second section includes a dropdown menu labeled "Select an NFT ID to Transfer." It is a <select> element that binds its value to the selectedNftId state variable and updates it using the onChange event handler. The dropdown options are dynamically generated from the sortedIndexes array.
There is a paragraph <p> element with two buttons:
- "Mint and Transfer NFT" button: It triggers the mintAndTransferNft function when clicked.
- If nftsAsBalanceCount is less than 2, the condition is false, and container-box-2 is not rendered.
To put it together, this is how the code would look like, additionally there is explanation to whats happening in these two conditions :
- The sortedIndexes variable is assigned the sorted array nftIndexes using the sort method, sorting the indexes in ascending order.
- The code returns a JSX structure that consists of a container (<div className="container">) containing two container boxes (container-box-1 and container-box-2), which are conditionally rendered based on the value of nftsAsBalanceCount.
- container-box-1 displays different content based on the condition nftsAsBalanceCount >= 2:
If the condition is true, it renders a <div> element containing the following elements:
- A paragraph <p> element displaying the message "You have {nftCount} NFTs in your balance," where {nftCount} is the value of the nftCount variable.
- Two buttons: "Mint NFTs as balance" and "View minted on SCW address on OpenSea." The first button triggers the mintNftAsBalance function when clicked, and the second button opens a new window with the URL specified in nftURL when clicked.
If the condition is false, it renders a paragraph <p> element displaying the message "Please Mint more NFTs to add as balance for transfer" and a "Mint NFTs as balance" button, which triggers the mintNftAsBalance function when clicked.
- container-box-2 is conditionally rendered only if nftsAsBalanceCount >= 2. It displays a <div> element with the className="container-box-2". Inside this div, there are the following elements:
- A <label> element with the text "Recipient Address :" and an <input> element for entering the recipient's address. The value of the input is bound to the recipientAddress state variable, and its value updates using the onChange event handler.
- A dropdown menu labeled "Select an NFT ID to Transfer" (<select>) that displays options generated from the sortedIndexes array. The value of the dropdown is bound to the selectedNftId state variable, and it updates using the onChange event handler.
- A paragraph <p> element with two buttons:
- "Mint and Transfer NFT" button triggers the mintAndTransferNft function when clicked.
- "View Transferred NFTs on OpenSea" button opens a new window with the URL specified in transferURL when clicked.
- Finally, the component Minter is exported as the default export.
Overall, the Minter component provides a user interface that allows users to mint NFTs, view minted NFTs on OpenSea, specify a recipient address, select an NFT ID to transfer, and view transferred NFTs on OpenSea. The UI rendering is conditionally based on the number of NFTs in the balance.
This is how the final output would look like :
.png)
Final Steps :
Once you are done with above steps, you can do npm run dev to run the dapp on localhost. Remember to edit the .env files as I had mentioned. The frontend .env file will include your API key from Biconomy Dashboard and the verified contract address. Your backend env file specific to your contract folder will have PRIVATE Key of your metamask account which should have some funds and API Key from POLYGONSCAN.
You can check out the entire link to the GitHub repository here : https://github.com/vanshika-srivastava/gasless-batched